Page 4: Matrix Exercises
CS559 Spring 2023 Sample Solution
Over the past few pages, you saw the connection between the math of transformations and the code we write to draw using Canvas. On this page, you’ll get to try writing some code to test it out.
Hint for some of these: remember that the canvas transform
allows you to specify an affine transformation as a matrix. It’s up to you to figure out what the matrix should be and put it into the 6 values that are passed to transform
. You may want to review the required readings to see the standard forms of the matrices. You can often figure out what the entries of the resulting matrix are using the methods in “Reading Matrices” on page 2, without having to build the matrix up from simple transformations.
In cases where you do need to figure out the transformations… The JavaScript Math library has a useful function that does a 2-argument arctangent - see Math.atan2
. Given x
and y
it tells you the angle to rotate to.
Box 1: Exercise 1, Two Dots with Translate, Rotate, and Scale
For this box, you should edit 04-04-01.js (for 04-04-01.html). The function you have to change is at the beginning of the file. Feel free to read the rest of the code - but please don’t change it.
Given two points, we want to map the square (0,0) - (10,10) such that the first point shows at the (0,0) corner, and the second point shows at the top right corner (10,0). In other words, the red dot should be at the corner close to the red square, and the black dot should stay close to the black square.
The square should remain a square - it can turn and stretch uniformly, but it should not reflect (notice that the insides of the square are not symmetric). Both points should have the same behavior. Each point should be able to rotate and scale the square, but not reflect it. When moving one point, the other point should stay in the same place.
I wrote some UI code that draws the square, and draw a red dot for the first point and a black dot for the second point, and lets you move the points around. All you need to do is fill in the function twoDots
in
04-04-01.js with the code that performs the transformation. It should consist of calls to context.translate
, context.rotate
, and context.scale
. I put in a context.translate
just to get you started. You can drag around the dots to make sure your code works (the graders will do it).
Here are some snapshots of what this should look like when it is working:
Box 04-04-01 Rubric (2 points total)
Box 2: Exercise 2, Two Dots with Transform
Now, do that again, but this time do it using context.transform
- compute the 6 numbers that need to be passed. Do this in the twoDots
in
04-04-02.js function (
04-04-02.html). I put some example values in that don’t work correctly. (The same example images above apply here.)
Box 04-04-02 Rubric (2 points total)
Box 3: Exercise 3, Two Dots a Little Harder
This is same as box 2 - except that the corner opposite the upper left (e.g., the 10,10 corner) should appear at the green dot. That is, the corner of the square closest to the green square should be close to the green dot. You may choose how you make the transformation - but make sure it keeps the square a square. The function to edit is twoDots
in
04-04-03.js (
04-04-03.html).
Here are some snapshots of what this should look like when it is working:
Box 04-04-03 Rubric (2 points total)
Box 4: Exercise 4, Convert to Transform
In the code for this box ( 04-04-04.html and 04-04-04.js), each of the squares in the top Canvas is placed by a sequence of rotate, translate, and scale commands.
In the bottom canvas, each square is transformed by a transform
- you need to match it. For each square, you need to figure out how to change the 6 parameters of the transform
function to put the square in the correct place. We’ve done this for square 2 already. Pay attention to the dots in the square.
Box 04-04-04 Rubric (5 points total)
Box 5: Exercise 5, Shear/Skew
Shear (sometimes called skew) is a fundamental linear transformation. It is discussed in the textbook, specifically FCG4 Chapter 6. Unfortunately, Canvas doesn’t have it.
Put “shear-x” transformation into the shearX
function in
04-04-05.js (
04-04-05.html). The code for the following Canvas calls that function to test it. Since the shearX
does nothing right now, you see squares here. If you implement things correctly, you should see sheared boxes. The test code does save and restore - you should make shearX
work like context.rotate
, except that it is a function not a method.
Here is what it should look like when you are done:
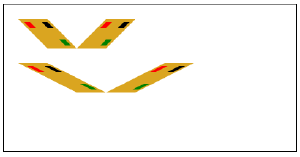
Hint: using context.transform
is the easiest way to do this.
Box 04-04-05 Rubric (3 points total)
Summary
That was a little math programming. Now on to Page 5 (Lots of Walking Objects).